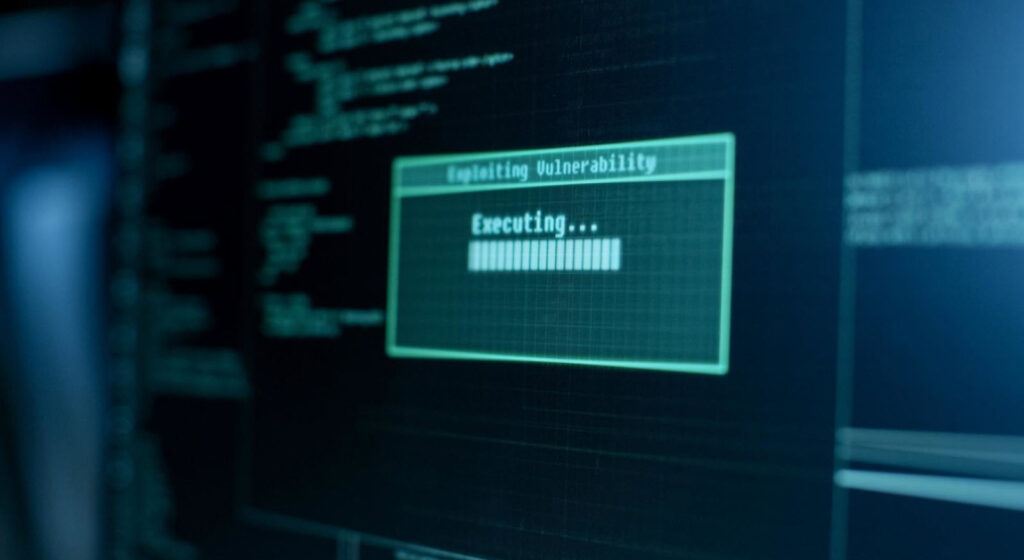
Exploit writing is a critical skill for penetration testers and cybersecurity professionals. It involves creating code that takes advantage of vulnerabilities in software to gain unauthorized access or control. While it sounds complex, understanding the basics can help you get started.
This guide will walk you through the steps of writing your first exploit.
What is an Exploit?
An exploit is a piece of code or a technique that takes advantage of a vulnerability in a system to gain unauthorized access or control. Exploits can be used to steal data, disrupt services, or gain control over a system. Writing an exploit involves understanding the vulnerability, crafting the payload, and delivering it to the target.
Prerequisites
Before you start writing exploits, you should have a basic understanding of the following:
- Programming Languages: Familiarity with languages like Python, C, or Ruby.
- Networking: Basic knowledge of how networks work, including protocols like TCP/IP.
- Operating Systems: Comfort with both Windows and Linux environments.
- Vulnerability Research: Understanding how to identify and analyze vulnerabilities.
Step-by-Step Guide to Writing an Exploit
Step 1: Identify the Vulnerability
The first step is to identify a vulnerability in the target software. This could be a buffer overflow, SQL injection, or any other type of vulnerability. Tools like Nessus, Nmap, or manual code review can help you find these vulnerabilities.
Step 2: Understand the Vulnerability
Once you’ve identified a vulnerability, you need to understand it thoroughly. This involves:
- Reading Documentation: Look at the software’s documentation to understand how it works.
- Analyzing Code: If you have access to the source code, analyze it to understand the vulnerability.
- Testing: Try to manually exploit the vulnerability to see how it behaves.
Step 3: Craft the Payload
A payload is the code that will be executed when the exploit is successful. The payload can be anything from a simple message to complex commands. Here’s a basic example of a payload in Python:
import socket
# Define the target IP and port
target_ip = '192.168.1.1'
target_port = 1234
# Create a socket object
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# Connect to the target
s.connect((target_ip, target_port))
# Send the payload
payload = b'\x41' * 100 # Example payload
s.send(payload)
# Close the connection
s.close()
Step 4: Deliver the Payload
The next step is to deliver the payload to the target. This could be through a network connection, a file upload, or any other method. The delivery method depends on the type of vulnerability and the environment.
Step 5: Test the Exploit
Once you’ve crafted and delivered the payload, you need to test the exploit. This involves:
- Running the Exploit: Execute your exploit code.
- Monitoring the Target: Check if the payload was successfully executed.
- Analyzing the Results: See if the exploit achieved the desired effect.
Step 6: Refine the Exploit
Based on your testing, you may need to refine the exploit. This could involve tweaking the payload, changing the delivery method, or adding error handling. The goal is to make the exploit more reliable and effective.
Example: Writing a Simple Buffer Overflow Exploit
Let’s walk through a simple example of a buffer overflow exploit in C.
Step 1: Identify the Vulnerability
Suppose you have a vulnerable C program that looks like this:
#include <stdio.h>
#include <string.h>
void vulnerable_function(char *str) {
char buffer[100];
strcpy(buffer, str);
printf("You entered: %s\n", buffer);
}
int main(int argc, char **argv) {
vulnerable_function(argv[1]);
return 0;
}
This program is vulnerable to a buffer overflow because it doesn’t check the length of the input string.
Step 2: Understand the Vulnerability
The `strcpy` function copies the input string into the `buffer` without checking its length. If the input string is longer than 100 characters, it will overflow the buffer.
Step 3: Craft the Payload
The payload will be a string longer than 100 characters. We can use Python to generate and send this payload.
import socket
# Define the target IP and port
target_ip = '127.0.0.1'
target_port = 1234
# Create a socket object
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# Connect to the target
s.connect((target_ip, target_port))
# Craft the payload
payload = b'A' * 100 + b'BBBB' # 100 A's followed by 4 B's
# Send the payload
s.send(payload)
# Close the connection
s.close()
Step 4: Deliver the Payload
In this example, the payload is sent over a network connection. You can run the Python script to deliver the payload to the vulnerable program.
Step 5: Test the Exploit
Run the vulnerable program and the Python script. The program should crash or behave unexpectedly, indicating a successful buffer overflow.
Step 6: Refine the Exploit
You may need to adjust the payload to achieve a specific effect, such as executing arbitrary code. This involves more advanced techniques like return address manipulation and shellcode injection.
Conclusion
Writing exploits is a complex but rewarding skill. By following these steps, you can start crafting your own exploits and understanding how vulnerabilities can be exploited. Remember, ethical hacking and penetration testing should always be conducted with permission and within legal boundaries.
Happy exploiting!